Initial SDK Setup
Initial SDK Setup
This walkthrough shows how to add Storyly to your Flutter application and show your first story in it.
You can also check out the demo on GitHub
Before you begin
This walkthrough contains sample instance information. However, if you want to work with your own content as well, please login into Storyly Dashboard and get your instance token.
The sample instance information for testing purposes;
eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJhY2NfaWQiOjc2MCwiYXBwX2lkIjo0MDUsImluc19pZCI6NDA0fQ.1AkqOy_lsiownTBNhVOUKc91uc9fDcAxfQZtpm3nj40
Installation
Storyly Flutter package is available through pub.dev. Source code is also available through the Storyly Flutter Github page, contributions are more than welcomed π
Please check the Installing tab of Storyly Flutter's pub.dev page for installing steps.
Tip
You can find the latest versionβs release notes here.
Android Installation
Storyly SDK is developed using Kotlin language. So, there are some requirements to use Kotlin plugin in the project. Especially if you're encountering a Plugin with id 'kotlin-android' not found.
error, this part fixes that.
You need to add kotlin-gradle-plugin
to Android project's main Gradle file (android/build.gradle
)
buildscript {
dependencies {
classpath("org.jetbrains.kotlin:kotlin-gradle-plugin:1.4.30")
}
}
Moreover, Storyly SDK is developed using the AndroidX library package instead of the Support Library. So, you need to ensure that your project also supports these packages. You can do this check and related lines if it's missing in the application's Android project's Gradle properties file (android/gradle.properties
)
android.useAndroidX=true
android.enableJetifier=true
Warning
Although Storyly SDK targets
Android API level 17 (Android 4.2, Jelly Bean)
or higher, PlatformView requires minimum APIAndroid API level 20 (Android 4.4W, KitKat)
. So, you need to update your app's minimum SDK version to 20.
Warning
Storyly SDK uses
Material Design Components
. So, you need to update your dependencies accordingly. You can check the official documentation for Enabling Material Components.
Warning
If your app only targets Android 21 or higher (minSdkVersion) then multidex is already enabled by default and you do not need the multidex support library.
However, if your minSdkVersion is set to 20 or lower, then you must use the multidex support library and make the following modifications to your app project:
android { defaultConfig { // ... multiDexEnabled true // ... } } dependencies { implementation 'com.android.support:multidex:2.0.1' }
iOS Installation
First of all, you need to update pods of the app; please do not forget to run pod update
.
// this should be executed inside 'ios' folder
pod update
Danger
Please note that you need to use Storyly with
use_frameworks!
option in Podfile. If you need to add it without usinguse_frameworks!
option, add following lines to yourPodfile
before updating Podsdynamic_frameworks = ['Storyly', 'SDWebImage'] pre_install do |installer| installer.pod_targets.each do |pod| if dynamic_frameworks.include?(pod.name) def pod.dynamic_framework?; true end def pod.build_type; Pod::BuildType.dynamic_framework end end end end
PlatformView's usage requires some updates on Info.plist
, add following lines:
<key>io.flutter.embedded_views_preview</key>
<true/>
How to edit Info.plist
In Xcode (or any editor), select the Info.plist from the Project Navigator. Right-click Open as -> Source Code. Now you will be able to add those two lines.
Warning
Storyly SDK targets
iOS 11
or higher.
Warning
Please note that CocoaPods version should be 1.9+
Import Storyly
Importing Storyly in your Flutter application is quite easy:
import 'package:storyly_flutter/storyly_flutter.dart';
Initialize StorylyView
Storyly
extends Widget
so that you can use inherited functionality as it is. You can add the following lines inside any view of your app;
Container(
height: 120,
child: StorylyView(
onStorylyViewCreated: onStorylyViewCreated,
androidParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN,
iosParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN
),
)
void onStorylyViewCreated(StorylyViewController storylyViewController) {
this.storylyViewController = storylyViewController;
// You can call any function after this via StorylyViewController instance.
}
Warning
storylyId : It's required for your app's correct initialization.
Tip
Please do not forget to use your own token. You can get your token from the Storyly Dashboard -> Settings -> App Settings
Just hit the run. Now, you should be able to enjoy Storyly
π!
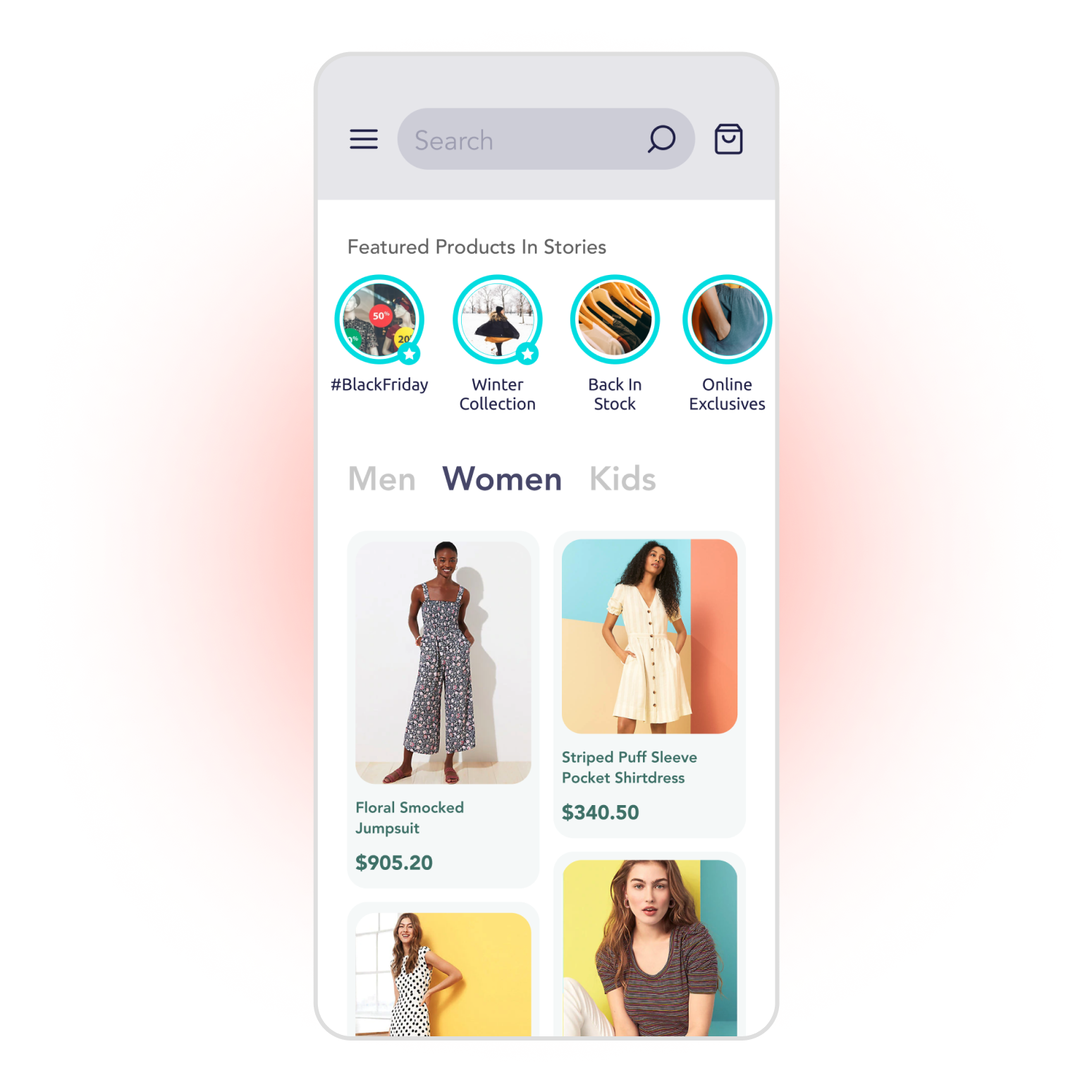
Warning
If you can't see Storlyly in your application, please check that your token is correct. For more details please check console logs.
Storyly Event Handling
This guide shows you how to handle Storyly events in your app. Storyly events provide insight on what is happening on a Storyly instance such as loading states, user redirections, user interaction.
Before you begin
You need to have the working Storyly integration as described in Initial SDK Setup.
Storyly Events
StorylyView widget notifies application when an event occurs. You can register the callback functions using the following code example. Moreover, you can chech the next sections to learn more about details of the events.
StorylyView(
...
storylyLoaded: (storyGroups, dataSource) => print("storylyLoaded"),
storylyLoadFailed: (errorMessage) => print("storylyLoadFailed"),
storylyActionClicked: (story) => print("storylyActionClicked"),
storylyEvent: (event, storyGroup, story, storyComponent) => print("storylyEvent"),
storylyStoryShown: () => print("storylyStoryShown"),
storylyStoryDismissed: () => print("storylyStoryDismissed"),
storylyUserInteracted: (storyGroup, story, storyComponent) => print("storylyUserInteracted"),
)
StorylyLoaded Event
This event will let you know that Storyly has completed its network operations, and the story group list has just been shown to the user. In order to notified about this event, use the following example:
StorylyView(
...
storylyLoaded: (storyGroups, dataSource) {
print("storylyLoaded")
},
)
Check storyGroupList
member of function parameter:
[
{
"id": 1,
"title": "...",
"index": 1,
"seen": true,
"iconUrl": "...",
"stories": [
{
"id": 1,
"title": "...",
"index": 1,
"seen": true,
"actionUrl": null,
}
]
}
]
StorylyLoadFailed Event
This event will let you know that Storyly has completed its network operations and had a problem while fetching your stories. In this case, users will see four empty story group icons, which we call skeleton view. In order to notified about this event, use the following example:
StorylyView(
...
storylyLoadFailed: (errorMessage) {
print("storylyLoadFailed")
},
)
Show/Hide Storyly
This guide shows use cases for showing or hiding Storyly bar in your app. To increase user experience when there are no stories available or stories are not loading using Storyly event handling.
You can also check out the demo on GitHub
Before you begin
You need to have the working Storyly setup as described in Initial SDK Setup.
Show Storyly
Use case for showing storyly if StorylyView is loaded and stories are available.
Create and initialize StorylyView with token from dashboard. storylyVisible
state is used for updating Visibility
.
For not to add already added StorylyView bar, check if initially load and storyGroupList size.
Event handling
storylyLoaded
event triggers first for available cached stories and second for request response with current stories.
void onStorylyLoaded(List<dynamic> storyGroupList) {
if (!storylyVisible && storyGroupList.length > 0) {
setState(() {
storylyVisible = true;
});
}
}
Warning
Visibility
parametermaintainState = true
for to StorylyView event handling to trigger when invisible.
Visibility(
visible: storylyVisible,
maintainState: true,
child: Container(
height: 120,
child: StorylyView(
androidParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN,
iosParam: StorylyParam()..storylyId = STORYLY_INSTANCE_TOKEN,
storylyLoaded: onStorylyLoaded,
storylyLoadFailed: onStorylyLoadFailed,
),
),
)
Hide Storyly
Use case for showing storyly if StorylyView is loaded and stories are added from dashboard.
Create and initialize StorylyView with token from dashboard.
Loading cached stories triggers storylyLoaded event before storylyLoadFailed event. Check for if cache loaded and storyGroupList size. If not loaded set storylyVisible
state to false
.
Event handling
storylyLoaded
event triggers first for available cached stories and later for up to date stories.
storylyLoaded
for cached stories will trigger beforestorylyLoadFailed
.
void onStorylyLoaded(List<dynamic> storyGroupList) {
if (!storylyVisible && storyGroupList.length > 0) {
storylyLoaded = true;
}
}
void onStorylyLoadFailed(String err) {
if (!storylyLoaded && storylyVisible) {
setState(() {
storylyVisible = false;
});
}
}
Warning
Visibility
parametermaintainState = true
for to StorylyView event handling to trigger when invisible.
Visibility(
visible: storylyVisible,
maintainState: true,
child: Container(
height: 120,
child: StorylyView(
androidParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN,
iosParam: StorylyParam()..storylyId = STORYLY_INSTANCE_TOKEN,
storylyLoaded: onStorylyLoaded,
storylyLoadFailed: onStorylyLoadFailed,
),
),
)
Test Mode
Before you begin
You need to have the working Storyly integration as described in Initial SDK Setup.
This guide shows how to show test groups created in Storyly Dashboard to the specific devices. The default value of storylyTestMode
is false, you need to explicitly define to set test devices.
Container(
height: 120,
child: StorylyView(
onStorylyViewCreated: onStorylyViewCreated,
androidParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN
..storylyTestMode = true,
iosParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN
..storylyTestMode = true,
),
)
Localization
This guide will walk you through the process of localizing all Storyly-related texts and content. You can deliver the appropriate content to each language/country pair by passing the locale
parameter on the client side.
To set the locale
, you need to use the IETF BCP 47 format as shown below:
Container(
height: 120,
child: StorylyView(
onStorylyViewCreated: onStorylyViewCreated,
androidParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN
.. storylyLocale = true,
iosParam: StorylyParam()
..storylyId = STORYLY_INSTANCE_TOKEN
.. storylyLocale = "tr-TR",
),
)
)
Tip
If you use the Translate option for Story Groups on the Storyly Dashboard, end user will see the content in their own
locale
.
Updated 4 months ago