Wishlist
Wishlist Feature allows your users to add items to their wishlist by simply clicking on the wishlist icon. After clicking on the icon an event will be triggered on the SDK, which will confirm the successful or the fail of addition of the item.
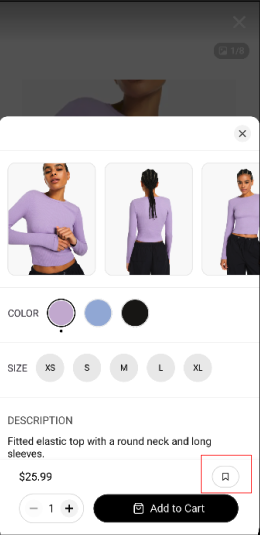
This walkthrough shows how to unlock the wishlist feature in your app.
Set Up Product Listener
This walkthrough shows you how to handle Wishlist events in your app. Wishlist events provide insight into what is happening on the Storyly SDK related to the products.
Before you begin
You need to have the working Vertical Feed integration as described in Initial SDK Setup
StorylyView
notifies the application when an event occurs. You can register the listener using the following code example and then override its functions to learn about specific events, which will be explained in the next sections.
storylyView.storylyProductListener = object : StorylyProductListener {
override fun storylyEvent(storylyView: StorylyView, event: StorylyEvent) {
}
To get notification about these basic events, you should override the following functions.
hydrateWishlist
This function allows you to hydrate your wishlist products.
UpdateWishlistEvent
This function will notify you about updates to the cart in a view
component.
onSuccess
It represents a callback function that will be executed if the "add to wishlist" operation is successful.
if (event == StorylyEvent.StoryWishlistAdded) {
onSuccess?.invoke(item?.copy(wishlist = true))
} else {
onSuccess?.invoke(item?.copy(wishlist = false))
}
It represents a callback function that will be executed if the "add to wishlist" or "remove from wishlist" operation is successful.
onFail
onFail?.invoke(STRWishlistEventResult("Insert Fail Message Here"))
It represents a callback function that will be executed if the "add to wishlist" or "remove from wishlist" operation fails.
Usage of verticalFeedUpdateWishlistEvent
storylyView.storylyProductListener = object : StorylyProductListener {
override fun storylyEvent(storylyView: StorylyView, event: StorylyEvent) {
}
override fun storylyHydration(
storylyView: StorylyView,
products: List<STRProductInformation>
) {
}
override fun storylyUpdateCartEvent(
storylyView: StorylyView,
event: StorylyEvent,
cart: STRCart?,
change: STRCartItem?,
onSuccess: ((STRCart?) -> Unit)?,
onFail: ((STRCartEventResult) -> Unit)?
) {
}
override fun storylyUpdateWishlistEvent(
storylyView: StorylyView,
item: STRProductItem?,
event: StorylyEvent,
onSuccess: ((STRProductItem?) -> Unit)?,
onFail: ((STRWishlistEventResult) -> Unit)?
) {
when (event) {
StorylyEvent.StoryWishlistAdded -> {
Log.d("Shopping", "Item Added to Wishlist")
//This event sent when a product is added.
}
StorylyEvent.StoryWishlistFailed -> {
Log.d("Shopping", "Item Removed from Wishlist")
//This event sent when a product is removed.
}
StorylyEvent.StoryWishlistFailed -> {
Log.d("Shopping", "Wishlist Failed")
}
}
if (event == StorylyEvent.StoryWishlistAdded) {
onSuccess?.invoke(item?.copy(wishlist = true))
} else {
onSuccess?.invoke(item?.copy(wishlist = false))
}
onFail?.invoke(STRWishlistEventResult("Insert Fail Message Here"))
}
}
}
Wishlist Hydrate Function
This function provides the list of products that will be used as wishlist items. It is the appropriate place to identify and return the user's wishlist products for hydration.
storylyView.storylyProductListener = object : StorylyProductListener {
override fun storylyEvent(storylyView: StorylyView, event: StorylyEvent) {
}
override fun storylyHydration(
storylyView: StorylyView,
products: List<STRProductInformation>
) {
storylyView.hydrateWishlist(products)
Updated 4 months ago