Shoppable Stories
Warning
That documentation for
Storyly SDK 3.0
and over. If you are usingStoryly Web SDK 2.x.x
and lower, you should follow through with that documentation.
Shoppable Stories enables your users to add products and services within the cart and checkout directly within the Story without leaving the Story watching experience.
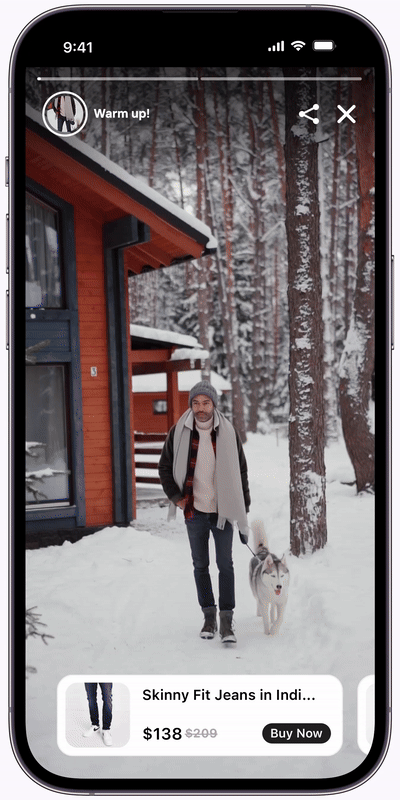
This walkthrough shows how to use Shoppable Stories in your app.
Before you begin
You need to have the working Storyly setup as described in Initial SDK Setup
Before you begin
You need to have at least 1 active Story with the Product Catalog in it to test this feature out.
Warning
In-Story Checkout step has not been implemented yet. Documentation will be updated once it becomes available.
Update Storyly Cart
storyCartUpdate Event
The storyCartUpdate
event handler is a callback function that gets triggered when a new product is added, a product from the cart is removed, or the cart is updated. You can define this event handler using the storylyWeb.on
function to customize the behavior when the cart is updated.
// Initialize Storyly with the storyCartUpdate event handler
storylyWeb.init({
token: token,
// ... other configuration option
});
storylyWeb.on('storyCartUpdate', handleStoryProductAdded);
// Define the storyCartUpdate event handler function
function handleStoryProductAdded(data) {
const { product, group, onError, onSuccess } = data;
console.log('New product added:', product);
// ... do something with the product, such as updating UI, triggering actions, etc.
onError({
message: `We're sorry to inform you that the item you're interested in purchasing is currently out of stock.`,
});
// Handle the onError newly added product based on your application's logic
onSuccess({
products: 'all products',
price: 'total_price',
sales_price: 'total_sales_price',
price_currency: 'currency',
});
// Update the cart with the product information accordingly
}
storyCartClicked Event
The storyCartClicked
method is an event that is triggered when a user clicks on the "Go to Cart" button in a product interactive. It is used to handle cart-related functionality in an application, such as navigating to the cart page, updating the UI, or performing other actions related to the clicked product.
storylyWeb.init({
token: token,
// Other configurations...
});
storylyWeb.on('storyCartClicked', storyCartClicked);
function storyCartClicked(data) {
// Access the product data from the 'data' parameter
const productData = data.products;
// ... do something with the product data, such as navigating to cart, triggering actions, etc.
}
Product Fallback for Hydration
There is a boolean parameter named isProductFallbackEnabled
which is used to determine whether the product data should be hydrated from the feed or not. By default, it is set to false, which means that product data will not be hydrated from the feed. However, when this parameter is set to true, product data will be hydrated from the feed.
You can set theisProductFallbackEnabled
to true
if you want to enable product fallback from the feed.
storylyWeb.init({
token: token,
props: {},
isProductFallbackEnabled: Boolean
});
Supplemental Product Feed Handling
If you're using a multi-market Product Feed depending on language or country, to automate the Shoppable Story Group creation, you can define the version of the feed that you'd like to use per user on the client side.
That way without creating Story Groups per each region or language, you can serve the relevant content to each market with a single Story Group. To set the locale
, you need to use the IETF BCP 47 format as shown below:
storylyWeb.init({
token: token,
props: {},
locale: "en-GB" / "es-ES" / "pt-PT" / "tr-TR" / "he-IL"
});
Storyly Cart
There is a boolean parameter named isProductCartEnabled
which adds cart icon to top of the left of Shoppable Stories. By default, it is set to false, but, when this parameter is set as true, the cart and the cart icon will be enabled on Shoppable Stories.
storylyWeb.init({
token: token,
props: {},
isProductCartEnabled: Boolean
});
No Cart Integration
If there is no cart integration, you can use the storyNoCartIntegration
event to handle call to actions of product.
storylyWeb.on('storyNoCartIntegration', (product) => {
// Triggered when called to action of product.
});
You can reach the product URL under product datas.
Product Hydration
The storylyHydration
function is a callback event that is triggered when the product data has been successfully retrieved from Stories. It provides you with an array of objects representing the found product IDs in the Stories.
To use the storylyHydration
function, define the storylyHydration
callback function in the storylyWeb.on
method, which will be triggered when the product data has successfully been retrieved from Stories.
Inside the storylyHydration
callback, you can access productIds
, which represents the found product IDs in the Stories. Perform any necessary actions with the product IDs, such as processing product data for hydration.
storylyWeb.init({
token: token,
});
storylyWeb.on('storylyHydration', (products: [Object] => {
// Triggered when called to action of product.
console.log(products);
});
Parameters
products
(Array of objects): An array of objects representing the hydrated product data from stories. Each object contains the following properties:id
(String): The unique identifier for the product.product_group_id
(String): The identifier for the product group to which the product belongs.product_id
(String): The identifier for the product.
setStorylyHydration Method
The setStorylyHydration
function allows you to hydrate your products in Storyly. It takes a list of products as input and updates the hydration status of these products in Storyly.
To use the setStorylyHydration
function, you need to provide a list of products as input. The list of products should be an array of objects, where each object represents a product.
// Call the hydrateProducts function with the products data
storylyWeb.setStorylyHydration(products);
Here is an example of asetStorylyHydration
. We set the product data manually as an example, but you can set your data from your database as well.
// Define the products data to hydrate
const products = [
{
id: '1014-67-1008',
account_id: 1014,
feed_id: 67,
product_id: '1008',
product_group_id: '1005',
title: 'Title',
description: "Description",
url: 'https://www.storyly.io/',
mobile_url: '',
image_urls: [
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
],
price: 0,
sales_price: 79.99,
price_date: '',
price_currency: 'USD',
unit_pricing_measure: '',
availability: 'in stock',
category: '',
type: '',
brand: '',
condition: '',
age_group: '',
gender: '',
variant: [],
},
// ... add more product objects as needed
];
// Call the hydrateProducts function with the product data
storylyWeb.setStorylyHydration(products);
Warning
For a Story that needs product data, if that product data is not provided, the Story Group containing that Story is not displayed.
Please make sure that you pass the necessary product data to avoid any issue.
Client-Side Automation
This functionality enables the generation of dynamic, interactive Stories tailored for users without the necessity of uploading a feed to the Storyly Dashboard.
First of all, you need to create a Shoppable Story Group and set the Story Group Client ID for the related Story Group on the Storyly Dashboard.
What you need to do is follow these steps:
- Go to the Content Page and create a New Story Group
- Choose the Create Shoppable Stories option
- Choose the Create Using Mobile Data option and then complete the next steps and create the Story Group
For the detailed steps, you can follow the Client-Side Automated Shoppable Stories user guide.
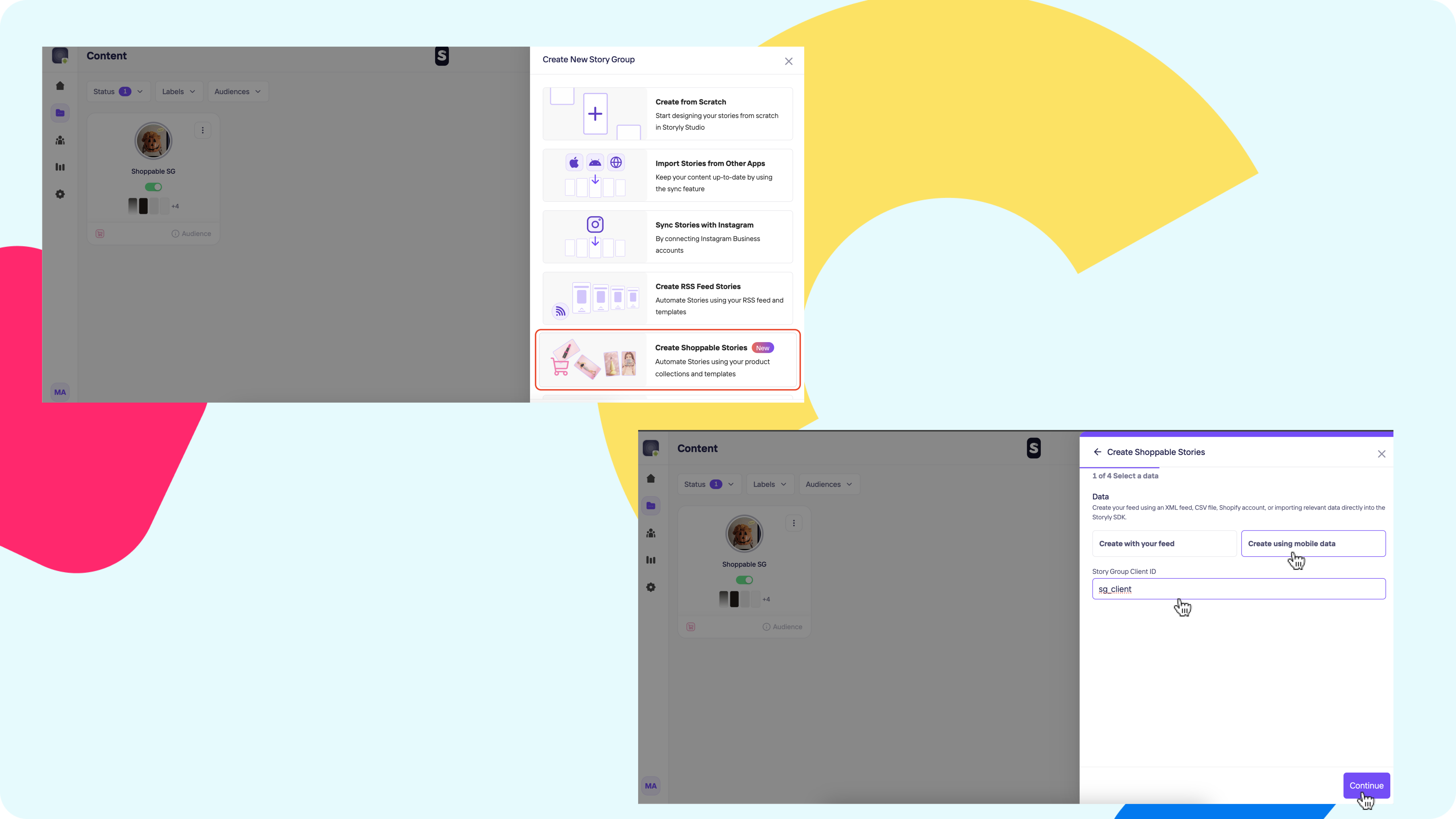
Tip
Story Group Client ID
is string value that you can set it on the Storyly Dashboard for each Story Group as a string. TheStory Group Client ID
must be a unique value for each Story Group.
Warning
Each
Story Group Client ID
corresponds to an array where each item represents a Story. These items contain the specific values assigned to attributes used within the Story Template.So we can consider the array of
Story Group Client ID
as a Story Group.
You should pass dynamic product information to Storyly SDK with the productFeed
method on the client side as shown below.
- First, you need to get your product information ;
const productFeed = {
sg_client: [
{
product_id: '1009',
product_group_id: '1005',
title: 'Title 1',
description: 'Description 1',
url: 'https://www.storyly.io/',
image_urls: [
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
],
price: 100,
sales_price: 79.99,
price_currency: 'USD',
availability: 'in stock',
category: '',
type: '',
discount: 10,
gender: '',
variant: [],
},
{
product_id: '1010',
product_group_id: '1006',
title: 'Title 2',
description: 'Description 2',
url: 'https://www.storyly.io/',
image_urls: [
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
],
price: 100,
sales_price: 79.99,
price_currency: 'USD',
availability: 'in stock',
category: '',
type: '',
discount: 10,
gender: '',
variant: [],
},
],
sg_client_2: [{
product_id: '1011',
product_group_id: '1007',
title: 'Title 3',
description: 'Description 3',
url: 'https://www.storyly.io/',
image_urls: [
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
'https://random-feed-generator.vercel.app/images/cosmetics/group-5/1.jpg',
],
price: 100,
sales_price: 79.99,
price_currency: 'USD',
availability: 'in stock',
category: '',
type: '',
discount: 10,
gender: '',
variant: [],
}
]
};
- Then, you should pass product information to storyly-web SDK with the
productFeed
method like shown below.
storylyWeb.init({
token: TOKEN,
productFeed: productFeed
});
Updated 5 months ago