UI Customizations
This guide shows you how to customize the Storyly experience of your users. These customizations apply to Storyly Bar, Story Groups, and Stories.
Before you begin
It is advised to apply these customizations after
StorylyInit
if you are planning to do the customizations programmatically.
Storyly Bar Customizations
This section shows supported customizations on the Storyly bar.
Story Bar Styling
This styling changes the orientation of Story Groups, the number of rows/columns (sections), the horizontal and vertical distance between the Story Groups, and the edge paddings of the first and last Story Groups.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setBarStyling(
styling: StorylyBarStyling.Builder()
.setOrientation(orientation: StoryGroupListOrientation)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setBarStyling(
styling: StorylyBarStyling.Builder()
.setSection(count: Int)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setBarStyling(
styling: StorylyBarStyling.Builder()
.setHorizontalEdgePadding(padding: Int)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setBarStyling(
styling: StorylyBarStyling.Builder()
.setVerticalEdgePadding(padding: Int)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setBarStyling(
styling: StorylyBarStyling.Builder()
.setHorizontalPaddingBetweenItems(padding: Int)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setBarStyling(
styling: StorylyBarStyling.Builder()
.setVerticalPaddingBetweenItems(padding: Int)
.build()
)
.build()
)
Tip
Orientation: This is set as .Horizontal by default, if you'd like to have a vertical, grid-like view of Story Groups, you can set it as .Vertical.
Sections: Based on the orientation, it's the row count of Story Groups for horizontal orientation or column count of Story Groups for vertical orientation.
Horizontal Edge Padding: Edge padding value of the first and last Story Groups for horizontal orientation.
Vertical Edge Padding: Edge padding value of the first and last Story Groups for vertical orientation.
Horizontal Padding Between Items: Horizontal padding value between Story Groups.
Vertical Padding Between Items: Vertical padding value between Story Groups.
Warning
You should set pixel values for the variables of
StoryGroupListStyling
.
Storyly Layout Direction
This attribute changes the layout direction of the Storyly Bar and Story-watching experience. Storyly Bar can either be LTR (Left to right) or RTL (Right to left). If your UI is bidirectional and it changes depending on the device or app language you can use this attribute.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setLayoutDirection(direction: StorylyLayoutDirection)
.build()
)
Warning
Storyly will use the LTR layout if the
setLayoutDirection
is not set.
Story Group Customizations
This section shows supported customizations on the Story Groups.
Story Group Title Styling
This styling changes the visibility, font, text size, color, and number of lines of the Story Group title.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setTitleVisibility(isVisible: Bool)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setTitleSeenColor(color = Int)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setTitleNotSeenColor(color = Int)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setTitleTypeface(typeface: Typeface)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setTitleTextSize(typeSizePair: Pair<Int, Int>)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setTitleMinLineCount(count: Int?)
.build()
)
.build()
)
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setTitleMaxLineCount(count: Int?)
.build()
)
.build()
)
//Overrides min and max lines
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
setTitleLineCount(count: Int?)
.build()
)
.build()
)
Usage of setTitleTextSize
If you want set size as px, you can use this method as shown below;
.setTitleTextSize(typeSizePair = Pair(TypedValue.COMPLEX_UNIT_PX, 50))
If you want set size as dp, you can use this method as shown below;
.setTitleTextSize(typeSizePair = Pair(TypedValue.COMPLEX_UNIT_DIP, 50))
Story Group Icon Background Color
This function changes the background color of the Story Group icon that is shown to the user as a skeleton view till the Stories are loaded.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setIconBackgroundColor(color: Int)
.build()
)
.build()
)
Story Group Icon Border Seen State Color
This function changes the border color of the Story Group icons that are seen by the user. The border consists of color gradients.
Warning
At least 2 colors must be defined in order to use this attribute. If a single color is requested, a same color code can be used twice.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setIconBorderColorSeen(colors: List<Int>)
.build()
)
.build()
)
Story Group Icon Border Not Seen State Color
This function changes the border color of the Story Group icons that are unseen by the user. The border consists of color gradients.
Warning
At least 2 colors must be defined in order to use this attribute. If a single color is requested, a same color code can be used twice.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setIconBorderColorNotSeen(colors: List<Int>)
.build()
)
.build()
)
Story Group Pin Icon Color
If any of the Story Groups are selected as pinned groups from the dashboard, a little star icon will appear along with the Story Group icon. This function changes the background color of this pin icon.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setPinIconColor(color: Int)
.build()
)
.build()
)
Story Group Size
This function changes the size of the Story Group. Currently, supported sizes are Small
, Large
and Custom
sizes. The default Story Group size is Large
.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setSize(size: StoryGroupSize)
.build()
)
.build()
)
Tip
If you set your story group size as
Custom
, you can change a few measures of the story groups such as icon height, icon width, icon corner radius. Check the Custom Size Story Group Icon Styling.
Custom Size Story Group Icon Styling
This styling changes the shape of the Story Group icons and their corners. You can create square, circle, and oval-shaped icons using the following functions StorylyStoryGroupStyling
config.
Warning
This section is effective if you set your story group size as
Custom
. If you set any other size and use this attribute, your changes will not take effect.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setSize(StoryGroupSize.Custom)
.setIconHeight(height: Int)
.setIconWidth(width: Int)
.setIconCornerRadius(radius: Int)
.build()
)
.build()
)
Story Group Thematic Icon Image
This feature lets you use different Story Group images for different labels. If you set dark mode images for your Story Group from the Storyly dashboard, you can set a dark label to show these dark mode icons.
Warning
If you use the following methods to set the label without setting dark mode images from the dashboard, default group images will be used. If you already set the dark mode images but send a string label other than
dark
, again default group images will be used.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setIconThematicImageLabel(label: String?)
.build()
)
.build()
)
Story Group Animation
This attribute adds animation to the Story Group cover.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryGroupStyling(
styling: StorylyStoryGroupStyling.Builder()
.setIconBorderAnimation(animation: StoryGroupAnimation)
.build()
)
.build()
)
Warning
Story Group Animation is enabled by default. To disable it, you need to set it as
disabled
.
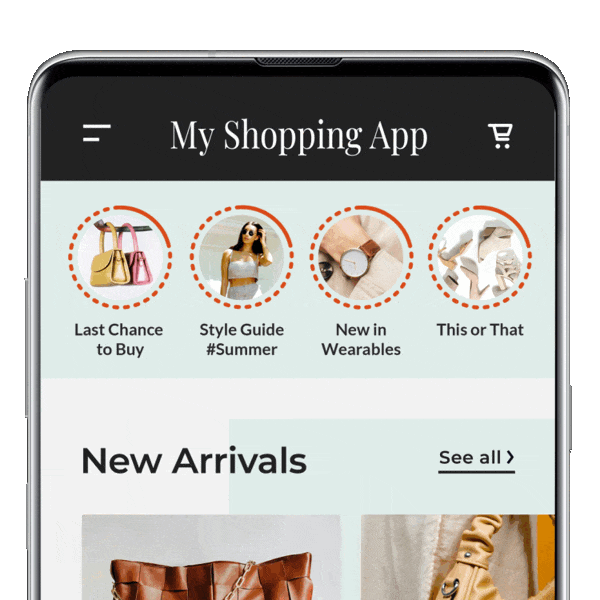
Warning
- Story Group animation applies to all Story Groups.
- Story Group animation is not available for Custom Story Group Styling.
Story Customizations
This section shows supported customizations on the Story view.
Story Title Color
This function changes the header text color of the Story view.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryStyling(
styling: StorylyStoryStyling.Builder()
.setTitleColor(color: Int)
.build()
)
.build()
)
Story Header Icon Border Color
This function changes the header icon border color of the Story view. The border consists of color gradients.
Warning
At least 2 colors must be defined in order to use this attribute. If a single color is requested, a same color code can be used twice.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryStyling(
styling: StorylyStoryStyling.Builder()
.setHeaderIconBorderColor(color: Array<Int>)
.build()
)
.build()
)
Story Progress Bar Color
This function changes the progress bar colors, two colors, of the Story view. The first defined color is the color of the background bars and the second one is the color of the foreground bars while watching the Stories.
Warning
Only 2 colors must be defined in order to use this attribute.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryStyling(
styling: StorylyStoryStyling.Builder()
.setProgressBarColor(color: [Array<Int>)
.build()
)
.build()
)
Story Header Icon and Title Visibility
This styling changes the visibility of the Story Group icon and title in Story view.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryStyling(
styling: StorylyStoryStyling.Builder()
.setTitleVisibility(isVisible: Bool)
.setHeaderIconVisibility(isVisible: Bool)
.build()
)
.build()
)
Story Close and Share Icon Styling
This styling changes the visibility and icon of the Story header close icon and share icon.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryStyling(
styling: StorylyStoryStyling.Builder()
.setCloseButtonIcon(icon: Drawable?)
.setShareButtonIcon(icon: Drawable?)
.build()
)
.build()
)
Story Title Font
This function changes the typeface of the Story view title.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryStyling(
styling: StorylyStoryStyling.Builder()
.setTitleTypeface(typeface: Typeface)
.build()
)
.build()
)
Storyly View Interactive Font
This function changes the typeface of all text view's font in interactive components.
storylyView.storylyInit = StorylyInit(
storylyId: STORYLY_INSTANCE_TOKEN,
config: StorylyConfig.Builder()
.setStoryStyling(
styling: StorylyStoryStyling.Builder()
.setInteractiveTypeface(typeface: Typeface)
.build()
)
.build()
)
Warning
Storyly will use Typeface.Default if custom font is not set.
Warning
Storyly will arrange font size for each text view in interactive components so that custom font size arrangement is not possible.
Custom Story Group Styling
Warning
This section is valid if you are using Storyly SDK version 1.16.0 or higher.
This guide shows you how to customize the Story Group view's style. Storyly provides three different pre-defined Story Group styles; Small
, Large
and Custom
. However, if you want to use a different style other than these pre-defined ones or if pre-defined ones do not satisfy your expectations then you should follow this section.
Warning
If you are using pre-defined sizes and want to apply only some customizations, follow UI Customizations section.
Story Group View Factory
You need to set storyGroupViewFactory
field of StorylyView
as a starting point of the setup. You need to create a class that is a subclass of StoryGroupViewFactory
and implement createView
function.
// Assume we have a StorylyView instance called storylyView
storylyView.storylyInit = StorylyInit(
storylyToken,
StorylyConfig.Builder()
.setStoryGroupStyling(
StorylyStoryGroupStyling.Builder()
.setCustomGroupViewFactory(SampleViewFactory(context))
.build()
)
.build()
)
class SampleViewFactory(val context: Context): StoryGroupViewFactory() {
override fun createView(): StoryGroupView {
return SampleView(context)
}
}
StoryGroupViewFactory
calls createView
function whenever a new view is required. It will request an instance of your view for each of the story groups while creating the StorylyView
. Therefore, each Story Group is associated with different instances of your view. Note that Storyly SDK uses RecyclerView to show lists so that a new view is not required for each time recycled views will be used.
Story Group View
StoryGroupView
class is used to represent the view that will be shown as a single Story Group. In order to use your own custom view, your view should be a subclass of StoryGroupView
class and implement its functions;
class SampleView(context: Context) : StoryGroupView(context) {
private val sampleViewBinding: SampleViewBinding = SampleViewBinding.inflate(LayoutInflater.from(context))
init {
addView(sampleViewBinding.root)
layoutParams = LayoutParams(LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT)
}
override fun populateView(storyGroup: StoryGroup?) {
// Handle populating view with Storyly related data
// ie, sampleViewBinding.sampleText.text = storyGroup?.title
}
}
populateView
function is called when your view is about to be shown. Its storyGroup
parameter has all of the information that you provided in the Storyly dashboard for your Story Groups. You can find the details of the StoryGroup
below:
data class StoryGroup(
val id: Int,
val title: String,
val iconUrl: String,
val thematicIconUrls: Map<String, String>?,
val coverUrl: String?,
val index: Int,
val seen: Boolean,
val stories: List<Story>,
val pinned: Boolean,
val type: StoryGroupType
)
Tip
You can find the explanation of each field from the API Reference section.
Tip
Please check the this github page for implemented samples.
Warning Additional data
Do not forget to use additional fields of the
storyGroup
parameter of thepopulateView
function. For instance, if you want to show pinned groups, you can usepinned
field of the object.
Summary
To sum up, in order to use your own custom group style, you need to follow the steps below:
- Create your own custom style in XML or programmatically.
- Use this style in a class that is a subclass of
StoryGroupView
by inflating the XML and adding its view or by adding views programmatically. - Override
populateView
function in the previous class you defined and by using the data instoryGroup
parameter, fill your UI components. - Create a subclass of
StoryGroupViewFactory
in order to serve as your factory. - Override
createView
function in the previous class you defined and return a new instance of yourStoryGroupView
subclass. - Create a new instance of the
StoryGroupViewFactory
subclass and setstoryGroupViewFactory
variable of the StorylyView with this instance.
Updated 4 days ago