Initial SDK Setup
Initial SDK Setup
This walkthrough shows how to add Storyly to your Android application and show your Vertical Video Feed in it.
Before you begin
Please login into Storyly Dashboard and get your widget token.
Installation
First, declare the dependency for the Storyly SDK in your app’s module Gradle file (usually app/build.gradle
).
android {
dependencies {
...
// You should add this line
implementation 'com.appsamurai.storyly:storyly:<latest-version>'
...
}
}
Tip
Please do not forget to replace
<latest-version>
. The latest version isYou can find the latest version’s release notes here.
Warning
Storyly SDK targets
Android API level 17 (Android 4.2, Jelly Bean)
or higher.
Warning
If your application targets devices that does not contain Google APIs, you need to initialize EmojiCompat class to use Emoji related features of Storyly such as Emoji and Rating Components. Otherwise, you will encounter a crash whenever you use any of these components in your Storyly widget.
Please follow Emoji Compat Bundled Fonts initialization steps to use Emoji features of Storyly.
Add Vertical Feed View
There are 2 view types available for Vertical Feed which are StorylyVerticalFeedBarView
and StorylyVerticalFeedView
.
As seen on the left-hand side, StorylyVerticalFeedBarView
is a bar-like design that enables users to scroll among available content horizontally.
As seen on the right-hand side, StorylyVerticalFeedView
is a grid-like design that enables users to scroll among available content vertically.
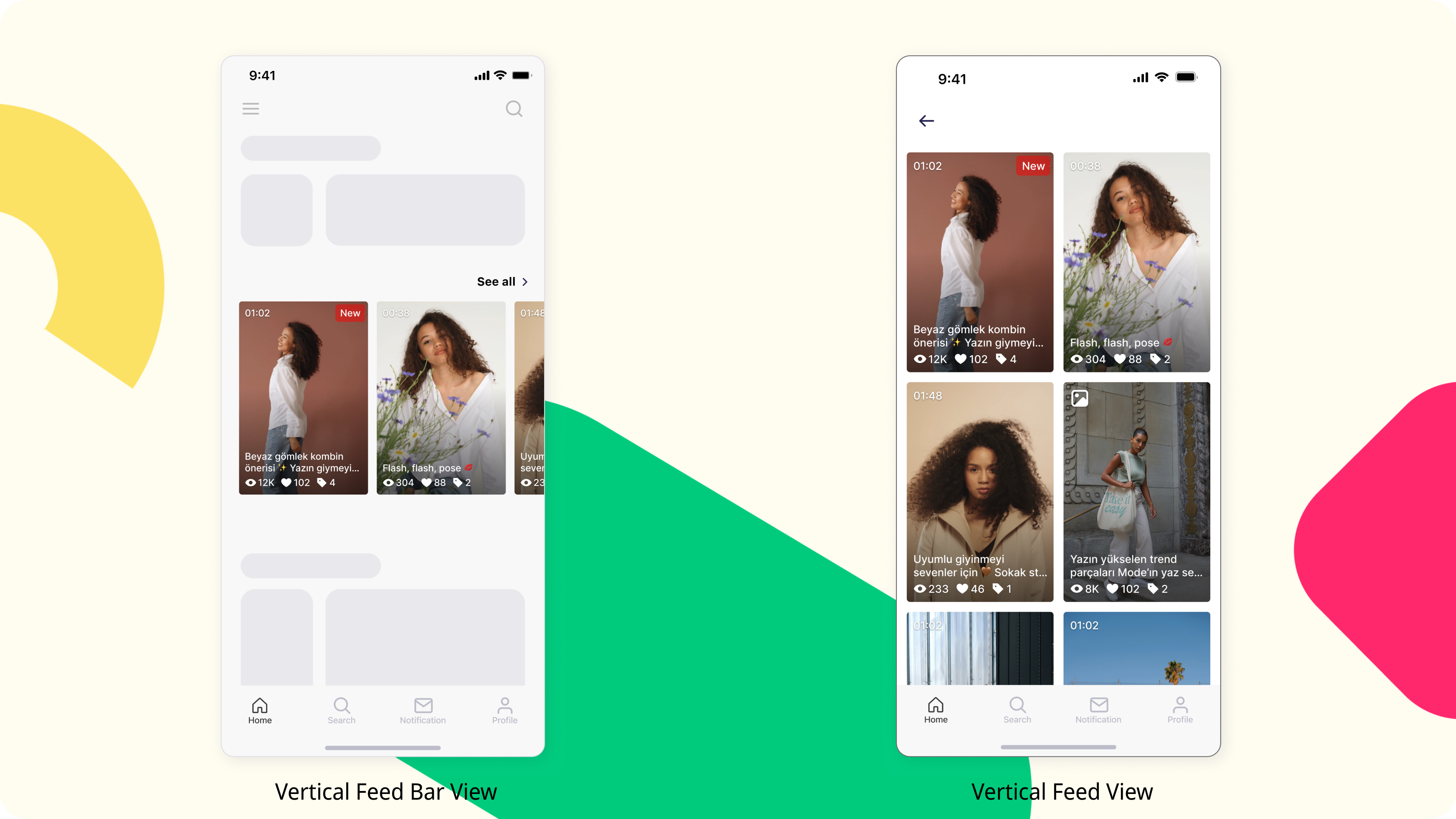
Warning
In the following sections of the documentation,
StorylyVerticalFeedView
is going to be used for code samples. However, the same applies while using the other view typeStorylyVerticalFeedBarView
.
You can add StorylyVerticalFeedView
to your app either from XML layout or using the programmatic approach.
StorylyVerticalFeedView
extends ViewGroup
so that you can use inherited functionality as it is. So, you can add StorylyVerticalFeedView
to any of the app’s layouts as a View component.
Adding from XML Layout
Open your XML layout and add these lines wherever you want to add StorylyVerticalFeedView
//Vertical Feed View
<com.appsamurai.storyly.StorylyVerticalFeedView
android:id="@+id/str_vertical_feed_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
//Vertical Feed Bar View
<com.appsamurai.storyly.StorylyVerticalFeedBarView
android:id="@+id/str_vertical_feed_bar_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
Programmatically
StorylyVerticalFeedView
extends ViewGroup
so that you can use inherited functionality as it is. So, you can initialize StorylyVerticalFeedView
using View’s constructors.
//Vertical Feed View
val StorylyVerticalFeedView = VerticalFeedView(this)
addView(StorylyVerticalFeedView)
//Vertical Feed Bar View
val StorylyVerticalFeedBarView = VerticalFeedView(this)
addView(StorylyVerticalFeedBarView)
//Vertical Feed View
StorylyVerticalFeedView verticalFeedView = new VerticalFeedView(this);
addView(StorylyVerticalFeedView);
//Vertical Feed Bar View
StorylyVerticalFeedBarView verticalFeedView = new VerticalFeedView(this);
addView(StorylyVerticalFeedBarView);
Initialize Vertical Feed View
You are one step away from enjoying Storyly. You just need to initialize StorylyVerticalFeedView
.
//Vertical Feed View
StorylyVerticalFeedView.storylyVerticalFeedInit = StorylyVerticalFeedInit(WIDGET_TOKEN)
//Vertical Feed Bar View
StorylyVerticalFeedBarView.storylyVerticalFeedInit = StorylyVerticalFeedInit(WIDGET_TOKEN)
//Vertical Feed View
StorylyVerticalFeedView.storylyVerticalFeedInit = StorylyVerticalFeedInit(WIDGET_TOKEN)
//Vertical Feed Bar View
StorylyVerticalFeedBarView.storylyVerticalFeedInit = StorylyVerticalFeedInit(WIDGET_TOKEN)
Tip
Please do not forget to use your own token. You can get your token from the Storyly Dashboard -> Settings -> App Settings
Just hit the run. Now, you should be able to enjoy the Vertical Feed experience🎉!
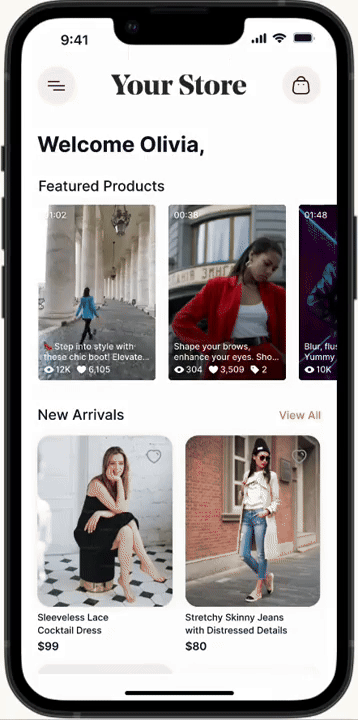
Warning
While initializing the
StorylyVerticalFeedView
, extra settings should be done withConfig
parameter. The aim is to gather all of the listed methods under theConfig
parameter.You can check the usage of
config
parameter as shown below and can check Configuration section for more details.While initializing the
StorylyVerticalFeedView
, extra settings should be done withConfig
parameter. The aim is to gather all of the listed methods under theConfig
parameter.
Configuration
While initializing the StorylyVerticalFeedView
, extra settings should be done with Config parameter. The aim is to gather all of the listed methods under the Config parameter.
Warning
Please check the relevant section for a detailed explanation.
StorylyVerticalFeedView.storylyVerticalFeedInit = StorylyVerticalFeedInit(
storylyId: storylyToken,
config: StorylyVerticalFeedConfig.Builder()
.setVerticalFeedBarStyling(
styling: StorylyVerticalFeedBarStyling.Builder()
.build()
)
.setVerticalFeedGroupStyling(
styling: StorylyVerticalFeedGroupStyling.Builder()
.build()
)
.setVerticalFeedStyling(
styling: StorylyVerticalFeedCustomization.Builder()
.build()
)
.setMaxItemCount(count: Int)
.setUserData(data: Map<String, String>)
.setLabels(labels: Set<String>?)
.setCustomParameter(parameter: String?)
.setLayoutDirection(StorylyLayoutDirection.LTR | .RTL)
.setTestMode(true)
.setLocale(locale: String?)
.setProductConfig(
config: StorylyProductConfig.Builder()
.build()
)
.setShareConfig(
StorylyShareConfig.Builder()
.setShareUrl(url: String)
.setFacebookAppID(id: String)
.build()
)
.build()
)
Set Up Listener
This walkthrough shows you how to handle Storyly events in your app. Storyly events provide insight into what is happening on a Storyly widget such as loading states.
Before you begin
You need to have the working Storyly integration as described in Initial SDK Setup
StorylyVerticalFeedView
notifies the application when an event occurs. You can register the listener using the following code example and then override its functions to learn about specific events, which will be explained in the next sections.
StorylyVerticalFeedView.verticalFeedListener = object : StorylyVerticalFeedListener {
// Override event functions
}
StorylyVerticalFeedView.setVerticalFeedListener(new StorylyVerticalFeedListener() {
// Override event methods
});
To get notification about these basic events, you should override the following functions in StorylyVerticalFeedListener
.
verticalFeedLoaded Event
This event will let you know that Storyly has completed its network operations, and the content list has just been shown to the user. To be notified about this event, use the following example:
override fun verticalFeedLoaded(view: StorylyVerticalFeedView,
feedGroupList: List<VerticalFeedGroup>,
dataSource: StorylyDataSource
@Override
public void verticalFeedLoaded(@NonNull StorylyVerticalFeedView view,
@NonNull List<VerticalFeedGroup feedGroupList,
@NonNull StorylyDataSource dataSource) {}
verticalFeedLoadFailed Event
This event will let you know that Storyly has completed its network operations and had a problem while fetching your content. In this case, users will see four empty covers, which we call the skeleton view. To be notified about this event, use the following example:
override fun verticalFeedLoadFailed(view: StorylyVerticalFeedView,
errorMessage: String) {}
@Override
public void verticalFeedLoadFailed(@NonNull StorylyVerticalFeedView view,
@NonNull String errorMessage) {}
verticalFeedActionClicked Event
This guide shows how to handle Action Button (CTA)
clicks from user.
When the end-user clicks on the an Action Button (CTA)
, redirection needs to be handled by the application itself. To handle this action, you must register StorylyVerticalFeedListener
and override verticalFeedActionClicked
function in it. You can register the listener using the following code example:
override fun verticalFeedActionClicked(view: StorylyVerticalFeedView
feedItem: VerticalFeedItem)
{
// verticalFeed.actionUrl is the field that contains the CTA URL
}
@Override
public void verticalFeedActionClicked(@NonNull StorylyVerticalFeedView view,
@NonNull verticalFeedItem feedItem)
{
// verticalFeed.media.actionUrl is the field that contains the CTA URL
}
});
How to Show/Hide Widget
This guide shows use cases for showing or hiding the widget in your app. To increase user experience when there is no content available or content is not loading using Storyly event handling.
Before you begin
You need to have the working Storyly setup as described in Initial SDK Setup
Show Widget
Use case for showing Storyly if StorylyVerticalFeedView
is loaded and content is available.
Create layout xml with added StorylyVerticalFeedView
. Initialize StorylyVerticalFeedView
with the token from the Dashboard.
Not to show the already visible StorylyVerticalFeedView
bar, check if initially loaded and verticalFeedGroupList
size. Set StorylyVerticalFeedView
visibility to View.VISIBLE
.
Event handling
verticalFeedLoaded
event triggers first for available cached content and second for request response with current stories. Detailed information about Set Up Listener
binding.StorylyVerticalFeedView.verticalFeedListener = object : StorylyVerticalFeedListener {
var initialLoad = true
override fun verticalFeedLoaded(view: StorylyVerticalFeedView,
feedGroupList: List<VerticalFeedGroup>) {
// for not to re-animate already loaded StorylyVerticalFeedView
if (initialLoad && feedGroupList.isNotEmpty()) {
initialLoad = false
StorylyVerticalFeedView.visibility = View.VISIBLE
}
}
}
StorylyVerticalFeedView.setVerticalFeedListener(new StorylyVerticalFeedListener() {
boolean initialLoad = true;??
@Override
public void verticalFeedLoaded(@NotNull StorylyVerticalFeedView view,
@NotNull List<VerticalFeedGroup> feedGroupList) {
// for not to re-animate already loaded StorylyVerticalFeedView
if (initialLoad && feedGroupList.size() > 0) {
initialLoad = false;
StorylyVerticalFeedView.setVisibility(View.VISIBLE);
}
}
}
Hide Widget
Use case for hiding Storyly if StorylyVerticalFeedView
is not loaded and content is not available.
Create layout xml with added StorylyVerticalFeedView
. Initialize StorylyVerticalFeedView
with the token from the Dashboard.
Not to show already visible StorylyVerticalFeedView
, check if initially loaded and verticalFeedGroupList
size. Set StorylyVerticalFeedView
visibility to View.VISIBLE
.
Loading cached content triggers verticalFeedLoaded
event before verticalFeedLoadFailed
event. Check for if the cache is loaded and verticalFeedGroupList
size. If the cache or request is not loaded, set the StorylyVerticalFeedView
visibility to View.INVISIBLE
or Visibility.GONE
.
Event handling
verticalFeedLoaded
event triggers first for available cached stories and later for up to date stories.
verticalFeedLoaded
for cached stories will trigger beforeverticalFeedLoadFailed
.
binding.StorylyVerticalFeedView.verticalFeedListener = object : StorylyVerticalFeedListener {
var verticalFeedLoaded = false
override fun verticalFeedLoaded(view: StorylyVerticalFeedView,
feedGroupList: List<VerticalFeedGroup>) {
if (feedGroupList.isNotEmpty()) {
verticalFeedLoaded = true
}
}
override fun verticalFeedLoadFailed(view: StorylyVerticalFeedView,
errorMessage: String) {
// if cached before not hide
if (!verticalFeedLoaded) {
StorylyVerticalFeedView.visibility = View.GONE;
}
}
}
StorylyVerticalFeedView.setVerticalFeedListener(new StorylyVerticalFeedListener() {
boolean verticalFeedLoaded = false;
@Override
public void verticalFeedLoaded(@NotNull StorylyVerticalFeedView view,
@NotNull List<VerticalFeedGroup> feedGroupList) {
if (feedGroupList.size() > 0) {
verticalFeedLoaded = true;
}
}
@Override
public void verticalFeedLoadFailed(@NotNull StorylyVerticalFeedView view,
@NotNull String errorMessage) {
// if cached before not hide
if (!verticalFeedLoaded) {
StorylyVerticalFeedView.setVisibility(View.GONE);
}
}
});
Set Up Product Listener
This walkthrough shows you how to handle Shoppable content events in your app. Shoppable events provide insight into what is happening on the Storyly widget related to products.
Before you begin
You need to have the working Storyly integration as described in Initial SDK Setup
StorylyVerticalFeedView
notifies the application when an event occurs. You can register the listener using the following code example and then override its functions to learn about specific events, which will be explained in the next sections.
StorylyVerticalFeedView.verticalFeedProductListener = object : StorylyVerticalFeedProductListener {
// Override event functions
}
StorylyVerticalFeedView.setVerticalFeedProductListener(new StorylyVerticalFeedProductListener() {
// Override event methods
});
To get notification about these basic events, you should override the following functions in StorylyVerticalFeedProductListener
.
verticalFeedUpdateCartEvent
This function will notify you about updates to the cart in a view
component.
STRCartItem
This class represents an individual item in the shopping cart. You can find the all properties of STRCartItem
here.
Info
You can get the
productId
,productGroupId
,price
,salesPrice
,currency
,desc
etc underchange.item
.
onSuccess
It represents a callback function that will be executed if the "update cart" operation is successful.
onSuccess?.invoke(STRCart(items = listof(),
oldTotalPrice = Float,
totalPrice = Float,
currency = String?
))
onFail
It represents a callback function that will be executed if the "update cart" operation fails.
onFail?.invoke(STRCartEventResult("Insert Fail Message here"))
Usage of verticalFeedUpdateCartEvent
StorylyVerticalFeedView.verticalFeedProductListener = object : StorylyVerticalFeedProductListener {
/**
* This function will notify you about updates the cart in a VerticalFeedView component
*
* view: StorylyVerticalFeedView widget in which the event is received
* event: event type which is received
* cart: Contains information about the items in the cart
* change: Represents the item being changed in the cart.
* onSuccess: It represents a callback function that will be executed if the "update cart" operation is successful
* onFail: It represents a callback function that will be executed if the "update cart" operation fails
*
*/
override fun verticalFeedUpdateCartEvent(
view: StorylyVerticalFeedView,
event: verticalFeedEvent,
cart: STRCart?,
change: STRCartItem?,
onSuccess: ((STRCart?) -> Unit)?,
onFail: ((STRCartEventResult) -> Unit)?,
) {
when (event){
verticalFeedEvent.VerticalFeedItemProductAdded -> {
Log.d("Shopping", "VerticalFeedItemProductAdded")
//This event sent when a product is added.
}
verticalFeedEvent.VerticalFeedItemProductUpdated -> {
Log.d("Shopping", "VerticalFeedItemProductUpdated")
//This event sent when a product is updated.
}
verticalFeedEvent.VerticalFeedItemProductRemoved -> {
Log.d("Shopping", "VerticalFeedItemProductRemoved")
//This event sent when a product is removed.
}
}
Log.d("Shoppable", "ShoppableEvent: ${event}")
Log.d("Shoppable", "ShoppableCart: ${cart}")
Log.d("Shoppable", "ShoppableChange: ${change}")
onSuccess?.invoke(STRCart(items = listof(),
oldTotalPrice = Float,
totalPrice = Float,
currency = String?
))
onFail?.invoke(STRCartEventResult("Insert Failed Message here"))
}
}
}
verticalFeedEvent
This function will notify you about all Vertical Feed related events so that you can make redirections accordingly. Also, you can send these events to your data platform to track user journeys on your end.
Usage of verticalFeedEvent
StorylyVerticalFeedView.verticalFeedProductListener = object : StorylyVerticalFeedProductListener {
override fun verticalFeedEvent(view: StorylyVerticalFeedView,
event: verticalFeedEvent) {
when (event){
verticalFeedEvent.VerticalFeedItemCheckoutButtonClicked -> {
Log.d("Shopping", "VerticalFeedItemCheckoutButtonClicked")
}
verticalFeedEvent.VerticalFeedItemCartButtonClicked -> {
Log.d("Shopping", "VerticalFeedItemCartButtonClicked")
}
verticalFeedEvent.VerticalFeedItemCartViewClicked -> {
Log.d("Shopping", "VerticalFeedItemCartViewClicked")
}
verticalFeedEvent.VerticalFeedItemProductSelected -> {
Log.d("Shopping", "VerticalFeedItemProductSelected")
}
}
}
}
StorylyVerticalFeedView.setVerticalFeedProductListener(new StorylyVerticalFeedProductListener() {
override fun verticalFeedEvent(
@NonNull view: StorylyVerticalFeedView,
@NonNull event: verticalFeedEvent) {
when (event){
verticalFeedEvent.VerticalFeedItemCheckoutButtonClicked -> {
Log.d("Shopping", "VerticalFeedItemCheckoutButtonClicked")
}
verticalFeedEvent.VerticalFeedItemCartButtonClicked -> {
Log.d("Shopping", "VerticalFeedItemCartButtonClicked")
}
verticalFeedEvent.VerticalFeedItemCartViewClicked -> {
Log.d("Shopping", "VerticalFeedItemCartViewClicked")
}
verticalFeedEvent.VerticalFeedItemProductSelected -> {
Log.d("Shopping", "VerticalFeedItemProductSelected")
}
}
}
});
VerticalFeedItemCheckoutButtonClicked Event
If isProductCartEnabled
is set to true, this event is sent when the "Go to Checkout" button is clicked.
VerticalFeedItemCartButtonClicked Event
This event is sent when the "Go to Cart" button is clicked from the success sheet.
VerticalFeedItemCartViewClicked Event
This event is sent when the cart icon on the content is clicked.
VerticalFeedItemProductSelected Event
This event is sent when the product is selected.
verticalFeedHydration
This function will notify you to get the products placed in Vertical Feed.
StorylyVerticalFeedView.verticalFeedProductListener = object : StorylyVerticalFeedProductListener {
override fun verticalFeedEvent(
view: StorylyVerticalFeedView,
event: verticalFeedEvent,
product: STRProductItem?,
extras: Map<String, String>
) {
/**
* This function will notify you to get the products placed in the Vertical Feed.
*
* - Parameter verticalFeedView: StorylyVerticalFeedView widget in which the user interacted with a component
* - Parameter productIds: Data class that represents the product information
*/
override fun verticalFeedHydration(view: StorylyVerticalFeedView,
products: List<STRProductInformation>) {
Log.d("Shopping", "verticalFeedHydration: ${products.toList()}")
}
}
}
Test Mode
Before you begin
You need to have the working Storyly integration as described in Initial SDK Setup
This guide shows how to show the test content created in Storyly Dashboard to the specific devices. The default value of isTestMode
is false, you need to explicitly define to set test devices.
StorylyVerticalFeedView.storylyVerticalFeedInit = StorylyVerticalFeedInit(WIDGET_TOKEN, isTestMode = true)
StorylyVerticalFeedView.storylyVerticalFeedInit(new StorylyVerticalFeedInit(WIDGET_TOKEN, isTestMode = true));
Localization
This guide will walk you through the process of localizing all Storyly-related texts and content. You can deliver the appropriate content to each language/country pair by passing the locale
parameter on the client side.
To set the locale
, you need to use the IETF BCP 47 format as shown below:
StorylyVerticalFeedView.storylyVerticalFeedInit = StorylyVerticalFeedInit(
storylyId: storylyToken,
config: StorylyVerticalFeedConfig.Builder()
.setLocale(locale: "tr-TR")
.build()
)
Tip
If you use the Localization feature on the Storyly Dashboard, end user will see the content in their own
locale
.
Updated 7 months ago